In this tutorial you will learn how to export gridview to pdf in asp.net using c#. Sometimes you may get requirement to export your gridview data to pdf format, so it is better that you should have idea to do so. Let's have a look over .aspx page that contains a gridview control
Export gridview to pdf in asp.net using c#
<asp:GridView ID="GridView1" runat="server" EnableTheming="false" AutoGenerateColumns="false" GridLines="None" Width="100%"> <Columns> <asp:TemplateField HeaderText="Sr#" HeaderStyle-Width="10%" HeaderStyle-HorizontalAlign="Center"> <ItemTemplate> <asp:Label ID="lblSrNo" runat="server" EnableTheming="false" Text='<%# Bind("SrNo")%>'></asp:Label> </ItemTemplate> <ItemStyle HorizontalAlign="Center" /> </asp:TemplateField> <asp:TemplateField HeaderText="First Name" HeaderStyle-Width="15%" HeaderStyle-HorizontalAlign="Left"> <ItemTemplate> <asp:Label ID="lblFirstName" runat="server" EnableTheming="false" Text='<%# Bind("FirstName")%>'></asp:Label> </ItemTemplate> <ItemStyle HorizontalAlign="Left" /> </asp:TemplateField> <asp:TemplateField HeaderText="Last Name" HeaderStyle-Width="15%" HeaderStyle-HorizontalAlign="Left"> <ItemTemplate> <asp:Label ID="lblLastName" runat="server" EnableTheming="false" Text='<%# Bind("LastName")%>'></asp:Label> </ItemTemplate> <ItemStyle HorizontalAlign="Left" /> </asp:TemplateField> <asp:TemplateField HeaderText="Result" HeaderStyle-Width="50%" HeaderStyle-HorizontalAlign="Left"> <ItemTemplate> <asp:Label ID="lblStatus" runat="server" EnableTheming="false" Text='<%# Bind("Status")%>'></asp:Label> </ItemTemplate> <ItemStyle HorizontalAlign="Left" /> </asp:TemplateField> </Columns> </asp:GridView> <asp:LinkButton ID="lnkExportPdf" runat="server" Text="Export to PDF" onclick="lnkExportPdf_Click"></asp:LinkButton>
As you have seen that we just have GridView Control and Link Button in our .aspx page. Using Link Button onClick event we will export the gridview to pdf. Let's have a look over how to do so
protected void lnkExportPdf_Click(object sender, EventArgs e) { //Total no of columns to be displayed int columnCount = 4; //Total no of records in gridview int rowCount = GridView1.Rows.Count; int tableRows = rowCount; //Creating Dynamic Table using iTextSharp namespace iTextSharp.text.Table grdTable = new iTextSharp.text.Table(columnCount, tableRows); //Giving Bordor to the Table grdTable.BorderWidth = 1; //Creating First Row that will contain the Title iTextSharp.text.Font fontIstRow = iTextSharp.text.FontFactory.GetFont(iTextSharp.text.FontFactory.HELVETICA, 8); iTextSharp.text.Cell c1 = new iTextSharp.text.Cell(""); c1.Add(new iTextSharp.text.Chunk("Student Result Card", fontIstRow)); c1.SetHorizontalAlignment("center"); c1.Colspan = 4; c1.Header = true; grdTable.AddCell(c1); c1.UseBorderPadding = false; //grdTable.BorderColor = System.Drawing.Color(); //Creating Sec Row that will contain 4 cells containing columns name iTextSharp.text.Font fontSecRow = iTextSharp.text.FontFactory.GetFont(iTextSharp.text.FontFactory.HELVETICA, 7); iTextSharp.text.Cell c2 = new iTextSharp.text.Cell(""); c2.Add(new iTextSharp.text.Chunk("Sr No.", fontSecRow)); c2.SetHorizontalAlignment("center"); grdTable.AddCell(c2); iTextSharp.text.Cell c3 = new iTextSharp.text.Cell(""); c3.Add(new iTextSharp.text.Chunk("First Name", fontSecRow)); c3.SetHorizontalAlignment("center"); grdTable.AddCell(c3); iTextSharp.text.Cell c4 = new iTextSharp.text.Cell(""); c4.Add(new iTextSharp.text.Chunk("Last Name", fontSecRow)); c4.SetHorizontalAlignment("center"); grdTable.AddCell(c4); iTextSharp.text.Cell c5 = new iTextSharp.text.Cell(""); c5.Add(new iTextSharp.text.Chunk("Result", fontSecRow)); c5.SetHorizontalAlignment("center"); grdTable.AddCell(c5); //Now creating Dynmaic Rows that will contain the gridview data iTextSharp.text.Font fontDynmaicRows = iTextSharp.text.FontFactory.GetFont(iTextSharp.text.FontFactory.HELVETICA, 6); for (int rowCounter = 0; rowCounter < rowCount; rowCounter++) { for (int columnCounter = 0; columnCounter < columnCount; columnCounter++) { string strValue = ""; if (columnCounter == 0) { if (GridView1.Rows[rowCounter].Visible == true) { Label SerialNo = GridView1.Rows[rowCounter].Cells[0].FindControl("lblSrNo") as Label; strValue = SerialNo.Text; iTextSharp.text.Cell c6 = new iTextSharp.text.Cell(""); c6.Add(new iTextSharp.text.Chunk(strValue, fontDynmaicRows)); c6.SetHorizontalAlignment("center"); grdTable.AddCell(c6); } } else if (columnCounter == 1) { if (GridView1.Rows[rowCounter].Visible == true) { Label FirstName = GridView1.Rows[rowCounter].Cells[1].FindControl("lblFirstName") as Label; strValue = FirstName.Text; iTextSharp.text.Cell c6 = new iTextSharp.text.Cell(""); c6.Add(new iTextSharp.text.Chunk(strValue, fontDynmaicRows)); grdTable.AddCell(c6); } } else if (columnCounter == 2) { if (GridView1.Rows[rowCounter].Visible == true) { Label LastName = GridView1.Rows[rowCounter].Cells[2].FindControl("lblLastName") as Label; strValue = LastName.Text; iTextSharp.text.Cell c6 = new iTextSharp.text.Cell(""); c6.Add(new iTextSharp.text.Chunk(strValue, fontDynmaicRows)); grdTable.AddCell(c6); } } else if (columnCounter == 3) { if (GridView1.Rows[rowCounter].Visible == true) { //strValue = GridView1.Rows[rowCounter].Cells[3].Text; //For Data Bound Field Label Status = GridView1.Rows[rowCounter].Cells[3].FindControl("lblStatus") as Label; strValue = Status.Text; iTextSharp.text.Cell c6 = new iTextSharp.text.Cell(""); c6.Add(new iTextSharp.text.Chunk(strValue, fontDynmaicRows)); grdTable.AddCell(c6); } } } } //Giving Cell Padding and Cell Spacing to the Dynamic Table grdTable.Cellpadding = 1; grdTable.Cellspacing = 1; iTextSharp.text.Document Doc = new iTextSharp.text.Document(); iTextSharp.text.pdf.PdfWriter.GetInstance(Doc, Response.OutputStream); Doc.Open(); Doc.Add(grdTable); Doc.Close(); Response.ContentType = "application/pdf"; Response.AddHeader("content-disposition", "attachment; filename=ViewResult.pdf"); Response.End(); }
Important Note:-
You must have to download the itextsharp.dll from internet to export the gridview data to pdf else you will get this error
The type or namespace name 'iTextSharp' could not be found (are you missing a using directive or an assembly reference?)
After downloading itextsharp.dll from internet you must place it to bin folder of your web project.
As you have seen the code is pretty simple, I just create a dynamic table that will contain all the gridview data and then that table will be exported to the pdf.
First of all I create the first row of the table and set its colspan property to 4 and set its heading as Student Result Card. After then I create second row that contains four cells, each cell contains column name. After creating second row I create dynamic rows by using nested for loops that will get data from gridview and append data to that dynamic rows.
And here's my output
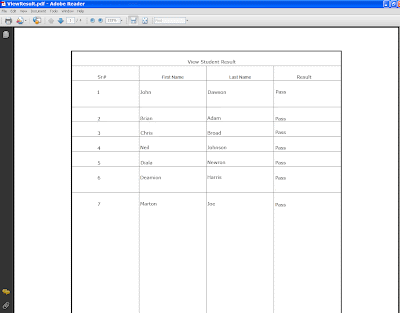
Happy Coding!!!